Using COMMANDS _MODULE PYTHON FOR MONITORING
- fredrickwer9
- Jan 28, 2019
- 1 min read
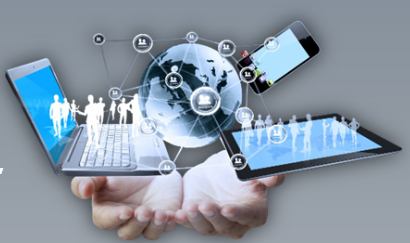
#!/bin/python
import commands import re import os import time
SERVERNAME = commands.getoutput("uname -n") status,output = commands.getstatusoutput("ls /app/documentum/wildfly9.0.1/server/DctmServer_MethodServer/deployments |grep deployed") lines = output.split('\n') for line in lines: if 'undeployed' in line: print("Undeployed files present in DctmServer_MethodServer") newpath = "undeployedmdfiles.txt" new_file = open(newpath, "w") new_file.write(line) print(line) print("Sending email notification and attachment of the file") commands.getoutput("uname -n >> undeployedmdfiles.txt") commands.getoutput('echo "Undeployed war files found on $HOSTNAME Server on MethodServer: manually fix please" >> undeployedmdfiles.txt') time.sleep(10) commands.getoutput("mail -s 'Undeployed WAR FILES Alert ON MethodServer' openlinux@yahoo.com < undeployedmdfiles.txt")
print("Cleaning up: ***************************************************") FILE = "undeployedmdfiles.txt" os.remove(FILE) time.sleep(1) print("Job completed: END") else: items = line.split(' ') print("Only Deployed files Present: DctmServer_MethodServer")
status2,output2 = commands.getstatusoutput("ls /app/documentum/wildfly9.0.1/server/DctmServer_WEBCACHE/deployments |grep deployed ") lines = output2.split('\n') for line in lines: if '.undeployed' in line: print("Undeployed files found in DctmServer_WEBCACHE") newpath1 = "undeployedwebcachefiles.txt" new_file = open(newpath1, "w") new_file.write(line) print(line) time.sleep(5) commands.getoutput("uname -n >> /app/documentum/fwerescripts/undeployedwebcachefiles.txt") commands.getoutput(' echo "Undeployed war files found on $HOSTNAME DctmServer_WEBCACHE: " >> undeployedwebcachefiles.txt') print("Sending email notification and attachment of the file") time.sleep(10) commands.getoutput("mail -s 'Undeployed WAR FILES Alert ON DctmServer_WEBCACHE' openlinux@yahoo.com < undeployedwebcachefiles.txt") time.sleep(5)
print("Cleaning up: ***************************************************") FILE = "undeployedwebcachefiles.txt" os.remove(FILE) time.sleep(1) print("Job completed: END") else: print("Only Deployed files Present on DctmServer_WEBCACHE")
Comments